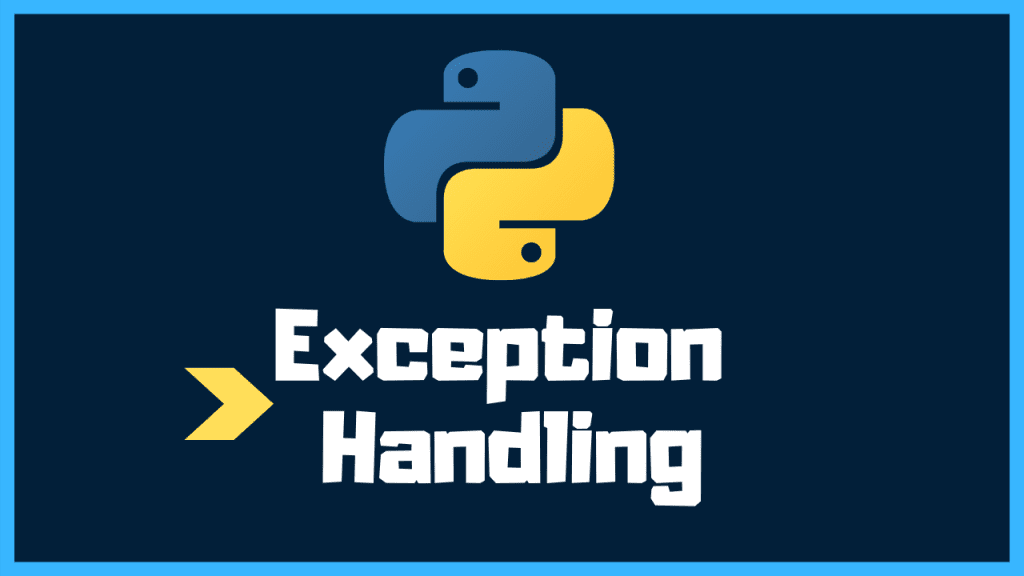
建议使用语义上适合具体的问题的 Exception 构造函数
例如:
raise ValueError('A very specific bad thing happened.')
Code language: JavaScript (javascript)
不要引发一般异常,避免抛出泛型Exception. 要捕获它,必须捕获所有其他将其子类化的更具体的异常。
例如:
def demo_bad_catch():
try:
raise ValueError('Represents a hidden bug, do not catch this')
raise Exception('This is the exception you expect to handle')
except Exception as error:
print('Caught this error: ' + repr(error))
>>> demo_bad_catch()
Caught this error: ValueError('Represents a hidden bug, do not catch this',)
Code language: PHP (php)
具体的捕获不会捕获一般异常:
def demo_no_catch():
try:
raise Exception('general exceptions not caught by specific handling')
except ValueError as e:
print('we will not catch exception: Exception')
>>> demo_no_catch()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 3, in demo_no_catch
Exception: general exceptions not caught by specific handling
Code language: PHP (php)
最佳实践:raise声明
请使用语义上适合具体问题 Exception 构造函数
raise ValueError('A very specific bad thing happened')
Code language: JavaScript (javascript)
这也方便地允许将任意数量的参数传递给构造函数:
raise ValueError('A very specific bad thing happened', 'foo', 'bar', 'baz')
Code language: JavaScript (javascript)
这些参数由Exception对象的args属性访问。例如:
try:
some_code_that_may_raise_our_value_error()
except ValueError as err:
print(err.args)
Code language: PHP (php)
打印
('message', 'foo', 'bar', 'baz')
Code language: JavaScript (javascript)
在 Python 2.5 中,添加了一个message
属性,BaseException
鼓励用户将 Exceptions
子类化并停止使用args
最佳实践:except 守则
当在except
子句中时,可能希望记录发生了特定类型的错误,然后重新引发。在保留堆栈跟踪的同时执行此操作的最佳方法是使用 raise
语句。例如:
logger = logging.getLogger(__name__)
try:
do_something_in_app_that_breaks_easily()
except AppError as error:
logger.error(error)
raise # just this!
# raise AppError # Don't do this, you'll lose the stack trace!
Code language: PHP (php)