Python 提供了各种库来处理Timestamp数据。例如,datetime 和time模块有助于处理多种日期和时间格式。除此之外,它还支持涉及Timestamp和时区的各种功能。
通过阅读本文,你将了解到:
- 如何在 Python中获取当前Timestamp
- 如何将Timestamp转换为datetime
- 如何将datetime转换为Timestamp
- 如何将Timestamp格式化为字符串对象,反之亦然
- 如何将带有偏移量的Timestamp对象转换为datetime对象
Python中的Timestamp是什么
Timestamp是 UNIX 中通常使用的时间形式,表示特定事件发生的时间。该信息可以精确到microseconds(微秒)。它对应于 datetime 实例的 POSIX Timestamp。
通常,日期和时间数据以 UNIX Timestamp格式保存。UNIX 时间或 Epoch 或 POSIX 时间是自 Epoch 以来的秒数。
Unix 时间(也称为纪元时间、POSIX 时间、纪元以来的秒数或 UNIX 纪元时间)描述了一个时间点,它是自 Unix 纪元以来经过的秒数,减去闰秒。
Unix 纪元是 1970 年 1 月 1 日(任意日期)的 00:00:00 UTC;闰秒被忽略,闰秒与它之前的秒具有相同的 Unix 时间,并且每一天都被视为恰好包含 86400 秒。
使用 UNIX 纪元时间作为 1970 年 1 月 1 日的原因是因为 UNIX 是在那个时间框架内开始使用的。
下图显示了如何以不同格式表示特定日期和时间
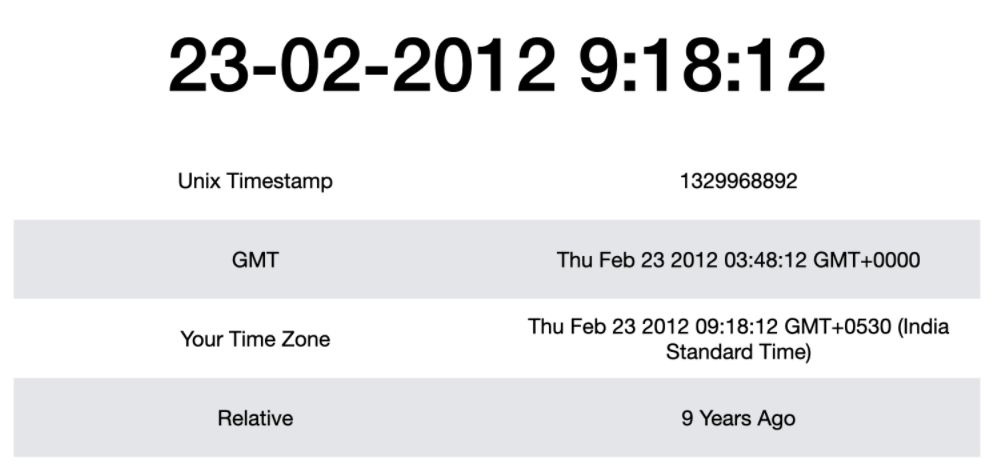
获取当前Timestamp
要在 Python 中获取当前Timestamp,请使用以下三个模块中的任何一个。
- datetime
- time
- calendar
datetime到Timestamp
datetime 模块的timestamp()
方法返回与 datetime 实例对应的 POSIX Timestamp。返回值为浮点数。
- 首先,使用Python 中的
datetime.now()
方法获取当前日期和时间。 - 接下来,将当前的时间传递给
datetime.timestamp()
方法获取 UNIX Timestamp
例子
from datetime import datetime
# Getting the current date and time
dt = datetime.now()
# getting the timestamp
ts = datetime.timestamp(dt)
print("Date and time is:", dt)
print("Timestamp is:", ts)
Code language: PHP (php)
输出:
Date and time is: 2021-07-03 16:21:12.357246
Timestamp is: 1625309472.357246
Code language: CSS (css)
注意:它返回浮点类型的Timestamp,如果需要获取整形数值的Timestamp,使用int(ts)构造函数将其转换为整数。
使用time模块获取Timestamp
time 模块的time()
方法 以Timestamp格式返回当前时间,它是从纪元时间 1970 年 1 月 1 日开始所经过的时间。
- 首先导入
time
模块 - 接下来,使用
time.time()
方法获取Timestamp
定义:此函数以浮点数形式返回自纪元以来的时间(以秒为单位)。
import time
# current timestamp
x = time.time()
print("Timestamp:", x)
# Output 1625309785.482347
Code language: PHP (php)
使用calendar模块获取Timestamp
使用calendar模块的calendar.timegm()
方法将当前时间转换为Timestamp。
- 首先,导入time和calendar模块
- 接下来,使用 time 模块的
time.gmtime()
方法获取 GMT 时间 - 最后,将其传递给
calendar.timegm()
方法获取Timestamp
示例:
import calendar
import time
# Current GMT time in a tuple format
current_GMT = time.gmtime()
# ts stores timestamp
ts = calendar.timegm(current_GMT)
print("Current timestamp:", ts)
# output 1625310251
Code language: PHP (php)
将Timestamp转换为datetime格式
虽然Timestamp的默认格式只是一个浮点数,但在某些情况下Timestamp将以 ISO 8601 格式表示。这看起来像下面带有 T 和 Z 字母的值。
2014-09-12T19:34:29Z
Code language: CSS (css)
这里的字母 T 代表时间,Z 代表零时区,它代表与协调世界时 (UTC) 的偏移量。
先看几个具有不同日期格式的示例。
我们可以使用datetime 模块中的fromtimestamp()
方法将Timestamp转换回datetime对象。
datetime.fromtimestamp(timestamp, tz=None)
它 返回 POSIX Timestamp对应的本地日期和时间,如 time.time()
.
如果未指定可选参数,则将Timestamp转换为平台的本地日期和时间,并且返回的 tz 对象
示例:
from datetime import datetime
# timestamp
ts = 1617295943.17321
# convert to datetime
dt = datetime.fromtimestamp(ts)
print("The date and time is:", dt)
# output 2021-04-01 22:22:23.173210
Code language: PHP (php)
将Timestamp转换为字符串
可以使用datetime格式转换Timestamp字符串。
- 首先,将Timestamp转换为datetime实例。
- 接下来,使用带有格式化方法的
strftime()
将Timestamp转换为字符串格式
它 返回 POSIX Timestamp对应的本地日期和时间,如time.time()
.
如果未指定可选参数,则将Timestamp转换为平台的本地日期和时间,并且返回的 tz 对象
示例:
from datetime import datetime
timestamp = 1625309472.357246
# convert to datetime
date_time = datetime.fromtimestamp(timestamp)
# convert timestamp to string in dd-mm-yyyy HH:MM:SS
str_date_time = date_time.strftime("%d-%m-%Y, %H:%M:%S")
print("Result 1:", str_date_time)
# convert timestamp to string in dd month_name, yyyy format
str_date = date_time.strftime("%d %B, %Y")
print("Result 2:", str_date)
# convert timestamp in HH:AM/PM MM:SS
str_time = date_time.strftime("%I%p %M:%S")
print("Result 3:", str_time)
Code language: PHP (php)
输出:
Result 1: 03-07-2021, 16:21:12
Result 2: 03 July, 2021
Result 3: 04PM 21:12
Code language: CSS (css)
以毫秒(Milliseconds)为单位获取Timestamp
datetime 对象带有Timestamp,而Timestamp又可以Milliseconds为单位显示。
示例:
from datetime import datetime
# date in string format
birthday = "23.02.2012 09:12:00"
# convert to datetime instance
date_time = datetime.strptime(birthday, '%d.%m.%Y %H:%M:%S')
# timestamp in milliseconds
ts = date_time.timestamp() * 1000
print(ts)
# Output 1329968520000.0
Code language: PHP (php)
获取 UTC Timestamp
可以从带有时区信息的 datetime 对象中获取Timestamp。我们可以使用timestamp()
方法将datetime对象转换为Timestamp
如果 datetime 对象是UTC 感知的,那么这个方法将创建一个UTCTimestamp。可以将 UTC 值赋给tzinfodatetime 对象的参数,然后调用该timestamp()方法。
示例datetime:从UTC 时区获取Timestamp
from datetime import datetime
from datetime import timezone
birthday = "23.02.2021 09:12:00"
# convert to datetime
date_time = datetime.strptime(birthday, '%d.%m.%Y %H:%M:%S')
# get UTC timestamp
utc_timestamp = date_time.replace(tzinfo=timezone.utc).timestamp()
print(utc_timestamp)
# Output 1614071520.0
Code language: PHP (php)
不同时区的datetime转换为Timestamp
我们已经看到如何从时区设置为timestamp的对象中获取信息datetimeUTC.
同样,,我们可以timestamp从时区与 UTC 不同的 datetime 对象获取信息。可以通过strptime()
偏移信息来完成。
from datetime import datetime
# Timezone Name.
date_String = "23/Feb/2012:09:15:26 UTC +0900"
dt_format = datetime.strptime(date_String, '%d/%b/%Y:%H:%M:%S %Z %z')
print("Date with Timezone Name::", dt_format)
# Timestamp
timestamp = dt_format.timestamp()
print("timestamp is::", timestamp)
Code language: PHP (php)
输出
Date with Timezone Name:: 2012-02-23 09:15:26+09:00
timestamp is:: 1329956126.0
Code language: CSS (css)
将整数Timestamp转换为datetime
可以使用相同的fromtimestamp()
或者 utcfromtimestamp()
方法将整数中的Timestamp值转换为对象。
示例:
import datetime
timestamp_int = 1329988320000
date = datetime.datetime.utcfromtimestamp(timestamp_int / 1e3)
print("Corresponding date for the integer timestamp is::", date)
Code language: JavaScript (javascript)
输出
Corresponding date for the integer timestamp is:: 2012-02-23 09:12:00
Code language: CSS (css)